Master VBA Day of Week Functions Easily
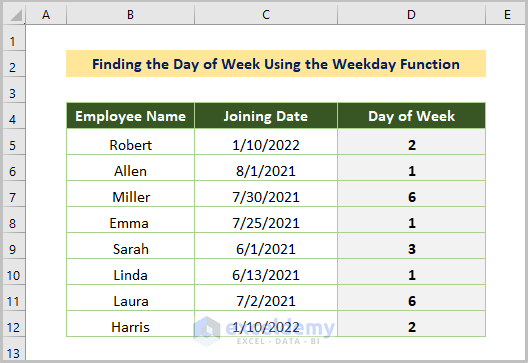
In the world of Excel automation, Visual Basic for Applications (VBA) stands as a powerful tool, enabling users to manipulate data with precision and efficiency. One common task that often arises is determining the day of the week for a given date. Whether you’re scheduling tasks, analyzing trends, or simply organizing data, mastering VBA day of week functions is essential. In this comprehensive guide, we’ll delve into the intricacies of VBA’s day of week functions, exploring their syntax, applications, and best practices.
Understanding the Basics
Before we dive into the specifics, let’s establish a foundational understanding of VBA’s date and time functions. VBA provides several built-in functions to work with dates, including Weekday
, WeekdayName
, and Format
. Each function serves a unique purpose, catering to different requirements.
The Weekday Function
The Weekday
function is perhaps the most straightforward method to determine the day of the week. Its syntax is as follows:
Weekday(date, [firstdayofweek])
- date: The date for which you want to find the day of the week.
- [firstdayofweek]: An optional parameter that specifies the first day of the week. If omitted, VBA defaults to
vbSunday
(1).
The function returns an integer value representing the day of the week, ranging from 1 (Sunday) to 7 (Saturday).
Example:
Dim myDate As Date
myDate = #1/15/2024#
MsgBox Weekday(myDate) ' Returns 3 (Tuesday)
Customizing the First Day of the Week
VBA allows you to customize the first day of the week using the firstdayofweek
parameter. This is particularly useful when working with international date formats or specific organizational requirements.
Constants for First Day of Week:
Constant | Value | Description |
---|---|---|
vbUseSystem |
0 | Use the system’s default first day of week |
vbSunday |
1 | Sunday (default) |
vbMonday |
2 | Monday |
vbTuesday |
3 | Tuesday |
vbWednesday |
4 | Wednesday |
vbThursday |
5 | Thursday |
vbFriday |
6 | Friday |
vbSaturday |
7 | Saturday |
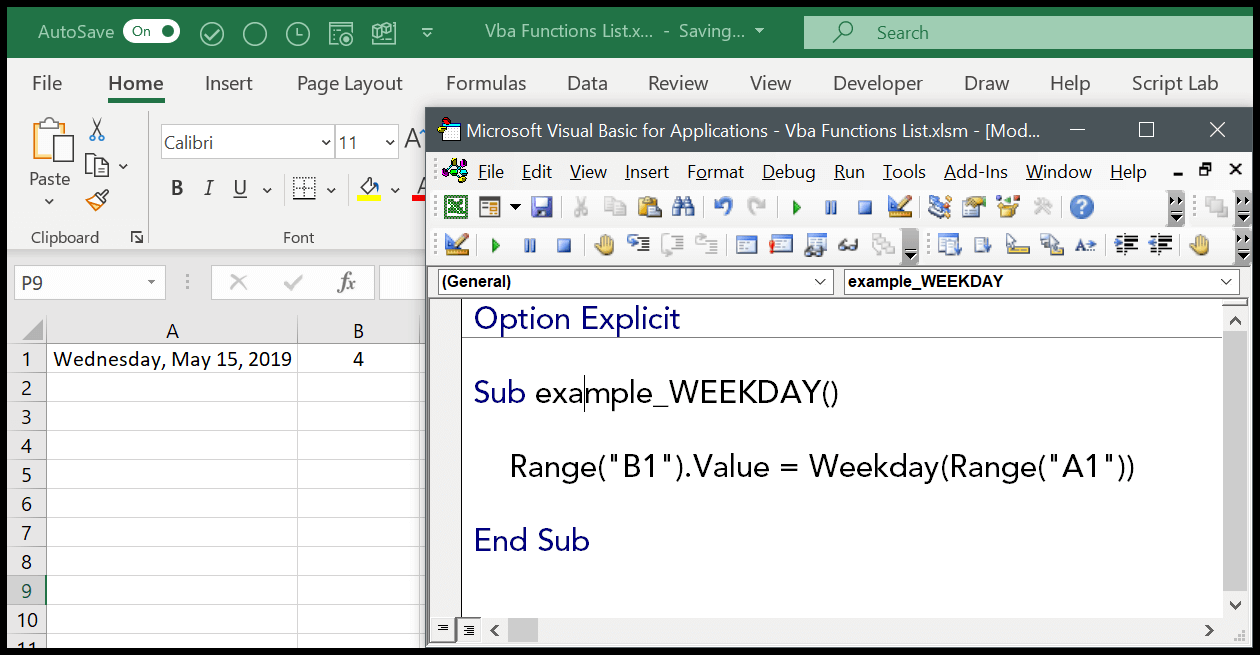
Example:
Dim myDate As Date
myDate = #1/15/2024#
MsgBox Weekday(myDate, vbMonday) ' Returns 2 (Tuesday, considering Monday as the first day)
Advanced Techniques
As you become more proficient with VBA’s day of week functions, you’ll discover advanced techniques to streamline your workflows.
Using WeekdayName for Descriptive Output
While Weekday
provides a numerical representation of the day, WeekdayName
offers a more descriptive output. This function returns the name of the day as a string.
Syntax:
WeekdayName(weekday, [abbreviate], [firstdayofweek])
- weekday: The integer value representing the day of the week (1-7).
- [abbreviate]: An optional parameter to abbreviate the day name (e.g., “Mon” instead of “Monday”).
- [firstdayofweek]: An optional parameter to specify the first day of the week.
Example:
Dim myDate As Date
myDate = #1/15/2024#
MsgBox WeekdayName(Weekday(myDate)) ' Returns "Tuesday"
Combining Functions for Complex Scenarios
In real-world applications, you may encounter scenarios that require combining multiple functions to achieve the desired result. For instance, you might need to format a date string that includes the day of the week.
Example:
Dim myDate As Date
myDate = #1/15/2024#
MsgBox Format(myDate, "dddd, MMMM dd, yyyy") ' Returns "Tuesday, January 15, 2024"
When working with international date formats, consider using the Format
function with locale-specific settings to ensure accurate and culturally appropriate output.
Practical Applications
Now that we’ve covered the fundamentals, let’s explore some practical applications of VBA day of week functions.
Scheduling Tasks
One common use case is scheduling tasks based on the day of the week. For example, you might want to generate a report every Monday or send reminders on Fridays.
Example:
Sub ScheduleTask()
Dim today As Date
today = Date
If Weekday(today) = vbMonday Then
' Generate report
MsgBox "Generating Monday report..."
End If
End Sub
Data Analysis
When analyzing data, you may need to categorize records based on the day of the week. This can be achieved using the Weekday
function in combination with conditional statements.
Example:
Sub AnalyzeData()
Dim ws As Worksheet
Dim lastRow As Long
Dim i As Long
Set ws = ThisWorkbook.Sheets("Data")
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
For i = 2 To lastRow
Select Case Weekday(ws.Cells(i, 1).Value)
Case vbMonday To vbFriday
ws.Cells(i, 2).Value = "Weekday"
Case vbSaturday, vbSunday
ws.Cells(i, 2).Value = "Weekend"
End Select
Next i
End Sub
When working with large datasets, consider using array formulas or optimizing your code to minimize processing time and improve performance.
Best Practices and Tips
To ensure efficient and maintainable code, follow these best practices and tips:
- Use meaningful variable names: Choose descriptive names for your variables to enhance code readability and understanding.
- Avoid hardcoding values: Use constants or named ranges to store frequently used values, making your code more flexible and easier to update.
- Implement error handling: Use error handling techniques, such as
On Error
statements, to gracefully handle unexpected errors and prevent crashes. - Optimize performance: When working with large datasets, consider using array formulas, minimizing screen updating, and optimizing loops to improve performance.
Pros and Cons of Using VBA Day of Week Functions
- Pros:
- Efficient and accurate day of week calculations
- Customizable first day of week settings
- Integration with other VBA functions and Excel features
- Cons:
- Limited to Excel and VBA environments
- Requires programming knowledge and experience
- Potential for errors and bugs in complex scenarios
Frequently Asked Questions (FAQ)
How do I determine if a date is a weekend?
+You can use the Weekday
function in combination with a conditional statement to check if a date falls on a weekend. For example: If Weekday(myDate) = vbSaturday Or Weekday(myDate) = vbSunday Then
.
Can I use VBA day of week functions with international date formats?
+Yes, VBA supports international date formats. You can use the Format
function with locale-specific settings or customize the first day of the week using the firstdayofweek
parameter.
How do I handle errors when working with dates in VBA?
+Implement error handling techniques, such as On Error
statements, to gracefully handle unexpected errors. Additionally, validate user input and use defensive programming practices to prevent errors.
Can I use VBA day of week functions in Excel formulas?
+No, VBA functions cannot be used directly in Excel formulas. However, you can create custom functions (User-Defined Functions) in VBA and use them in Excel formulas.
How do I optimize VBA code for large datasets?
+To optimize VBA code for large datasets, consider using array formulas, minimizing screen updating, and optimizing loops. Additionally, use efficient algorithms and data structures to reduce processing time.
By mastering VBA day of week functions, you’ll unlock new possibilities for Excel automation and data manipulation. With the knowledge and techniques presented in this guide, you’ll be well-equipped to tackle complex scenarios and develop efficient, maintainable code. Remember to follow best practices, stay organized, and continuously refine your skills to become a VBA expert.
Step-by-Step Guide to Creating a Custom Day of Week Function
- Open the VBA editor (Alt + F11)
- Insert a new module (Insert > Module)
- Define a custom function using the
Function
keyword - Implement the desired logic using VBA day of week functions
- Test the function in Excel by calling it from a cell or another VBA procedure
As you continue to explore VBA and its capabilities, you’ll discover new ways to streamline your workflows and enhance your productivity. Happy coding!